Tutoriels Backend
Routing with React
We will implement the following functionality in our project:
- Routing
Routing is the mechanism that allows you to navigate from one page to another on a website.
React has the react-router-dom library
This will allow us to quickly and easily manage navigation through routing.
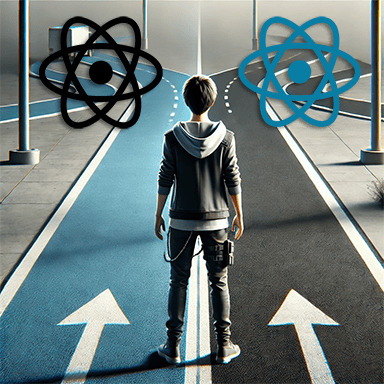
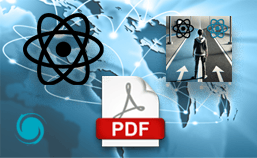
If you don't have time to read this entire guide,
download it now
Project creation
To be able to continue this tutorial we obviously need to have certain elements
- Node.js : The JavaScript platform
- Git : The version control software.
- Visual Studio code : A code editor.
You can check out the following tutorial which explains how to do it
In this tutorial we will use an existing project whose characteristics are
- Generated with create-react-app
# Create a demo directory (the name is arbitrary here)
mkdir demo
# Go to this directory
cd demo
# Get the source code on your workstation
git clone https://github.com/ganatan/angular-react-starter
# Go to the directory that was created
cd angular-react-starter
cd react
# Run the dependencies (or libraries) installation
npm install
# Run the program
npm run start
# Check its operation by launching the command in your browser
http://localhost:3000/
Initialization
React does not provide a module that deals with routing.
The documentation gives us the following advice
https://reactjs.org/docs/code-splitting.html#route-based-code-splitting
We will call an external library react-router-dom
The official website and the github repository are accessible at the following addresses
https://reacttraining.com/react-router/
https://github.com/ReactTraining/react-router
We need to install the corresponding dependency
# Installer
npm install --save react-router-dom
First we will create four files that will be used in routing.
- Home.js
- Contact.js
- About.js
- NotFound.js
import React from 'react'
const Home = () => (
<p>
home works!
</p>
)
export default Home
import React from 'react'
const Contact = () => (
<p>
contact works!
</p>
)
export default Contact
import React from 'react'
const About = () => (
<p>
about works!
</p>
)
export default About
import React from 'react'
const NotFound = () => (
<p>
NotFound works!
</p>
)
export default NotFound
Finally the routing processing will be done in the App.js file.
To complete, let's make a graphic modification in the App.css and Index.css files.
import React, { Component } from 'react';
import {
BrowserRouter as Router,
Route,
Link,
Switch,
} from 'react-router-dom'
import './App.css';
import Home from './Home';
import About from './About';
import Contact from './Contact';
import NotFound from './NotFound';
class App extends Component {
render() {
return (
<Router>
<div>
<header>
<section>
<h1> react-starter </h1>
<h2> (React version 18.0.2) </h2>
</section>
<nav>
<ul>
<li><Link to="/">Home</Link></li>
<li><Link to="/about">About</Link></li>
<li><Link to="/contact">Contact</Link></li>
</ul>
</nav>
</header>
<main>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
<Route exact path="*" component={NotFound} />
</Switch>
</main>
</div>
</Router >
);
}
}
export default App;
h1 {
color: blue;
}
body {
color: black;
font-weight: 400;
}
Conclusion
All that remains is to test the following scripts.
# développement
npm run start
http://localhost:3000/
# Tests
npm run test
# Compilation
npm run build
# Production
http-server -p 8080 -c-1 build
http://localhost:8080/
Code Source
The source code used at the beginning of the tutorial is available on github
https://github.com/ganatan/angular-react-starter
The source code obtained at the end of this tutorial is available on github
https://github.com/ganatan/angular-react-routing
How to create a From scratch application?
Create your ganatan account
Download your complete guides for free
Démarrez avec angular CLI 
Gérez le routing 
Appliquez le Lazy loading 
Intégrez Bootstrap 
Utilisez Python avec Angular 
Utilisez Django avec Angular 
Utilisez Flask avec Angular 
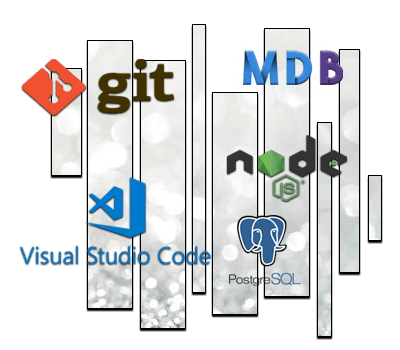
Share